Retrieving the first key in a Python dictionary is a handy skill for any programmer. It’s a straightforward process that involves accessing the keys of the dictionary and then getting the first one. By following these simple steps, you’ll be able to quickly and efficiently get the first key from any Python dictionary.
Step by Step Tutorial: Retrieving the First Key in a Python Dictionary
In this tutorial, we’ll go through the steps needed to retrieve the first key in a Python dictionary. This is a useful skill for when you want to access the initial element of a dictionary without having to loop through all the keys.
Step 1: Create a Dictionary
First, make sure you have a dictionary from which to retrieve the key.
Creating a dictionary in Python is simple. You can use curly braces {} with key-value pairs separated by commas. For example: my_dict = {'a': 1, 'b': 2, 'c': 3}
.
Step 2: Access the Dictionary’s Keys
Next, access the keys of the dictionary by using the keys()
method.
The keys()
method returns a view object that displays a list of all the keys. When you have this list, you can retrieve the first key by using indexing. Keep in mind that dictionaries are unordered collections, so "first" refers to the key that is returned first when iterating over the dictionary.
Step 3: Retrieve the First Key
Finally, retrieve the first key from the list of keys by using indexing.
To get the first key, you can convert the view object to a list and then access the first element using list indexing: list(my_dict.keys())[0]
.
After completing these steps, you’ll have successfully retrieved the first key from your Python dictionary. This can be useful for many programming tasks, such as iterating over items or accessing specific elements.
Tips: Retrieving the First Key in a Python Dictionary
- Keep in mind that dictionaries in Python versions before 3.7 are not ordered, so the first key may not always be the same.
- If you have a large dictionary, consider if you really need the first key, as converting keys to a list can be resource-intensive.
- Remember that the
keys()
method returns a view object, which is dynamic and reflects any changes to the dictionary. - Python dictionaries are inherently unordered until version 3.7, where they are ordered by insertion. However, relying on this order might not be the best practice for code compatibility.
- If you’re working with nested dictionaries, you’ll need to repeat this process for each level to retrieve the first key.
Frequently Asked Questions
What is a dictionary in Python?
A dictionary in Python is a collection of key-value pairs that is unordered, changeable, and indexed. It allows you to store and retrieve data efficiently.
Can you always retrieve the same first key from a dictionary?
In Python versions 3.7 and later, dictionaries maintain insertion order, so the first key will be consistent. In earlier versions, the first key may vary.
Is it possible to retrieve the first key without converting to a list?
In Python 3.7 and later, you can retrieve the first key using next(iter(my_dict))
without converting the keys to a list.
Can you use the popitem()
method to retrieve the first key?
The popitem()
method removes and returns the last inserted key-value pair in Python 3.7 and later, and an arbitrary pair in earlier versions. It’s not recommended for just retrieving the first key as it also modifies the dictionary.
What happens if the dictionary is empty?
If the dictionary is empty, trying to retrieve the first key will result in an error. Always check the size of the dictionary before attempting to get the first key.
Summary
- Create a dictionary.
- Access the dictionary’s keys.
- Retrieve the first key.
Conclusion
Retrieving the first key in a Python dictionary is a simple yet essential task that every programmer should know. Whether you’re a beginner or an experienced coder, understanding how to access the keys of a dictionary efficiently can save you time and enhance the performance of your code. With the straightforward steps outlined in this article, you’ll be able to accomplish this task with ease. Just remember to consider the version of Python you’re using since dictionaries are ordered by insertion from version 3.7 and onwards.
As with any coding practice, there are always nuances and exceptions to keep in mind. For instance, if you’re dealing with massive dictionaries or nested structures, you’ll need to adapt the basic principles to fit your specific use case. And, of course, the landscape of programming is always evolving, so staying updated with the latest Python releases and their features is crucial.
In conclusion, mastering the retrieval of the first key in a Python dictionary is just one of the many skills that make up a programmer’s toolkit. It’s a fundamental operation that may seem trivial at first glance but can have significant implications for the functionality and efficiency of your program. So go ahead, give it a try, and see how it can improve your Python projects!
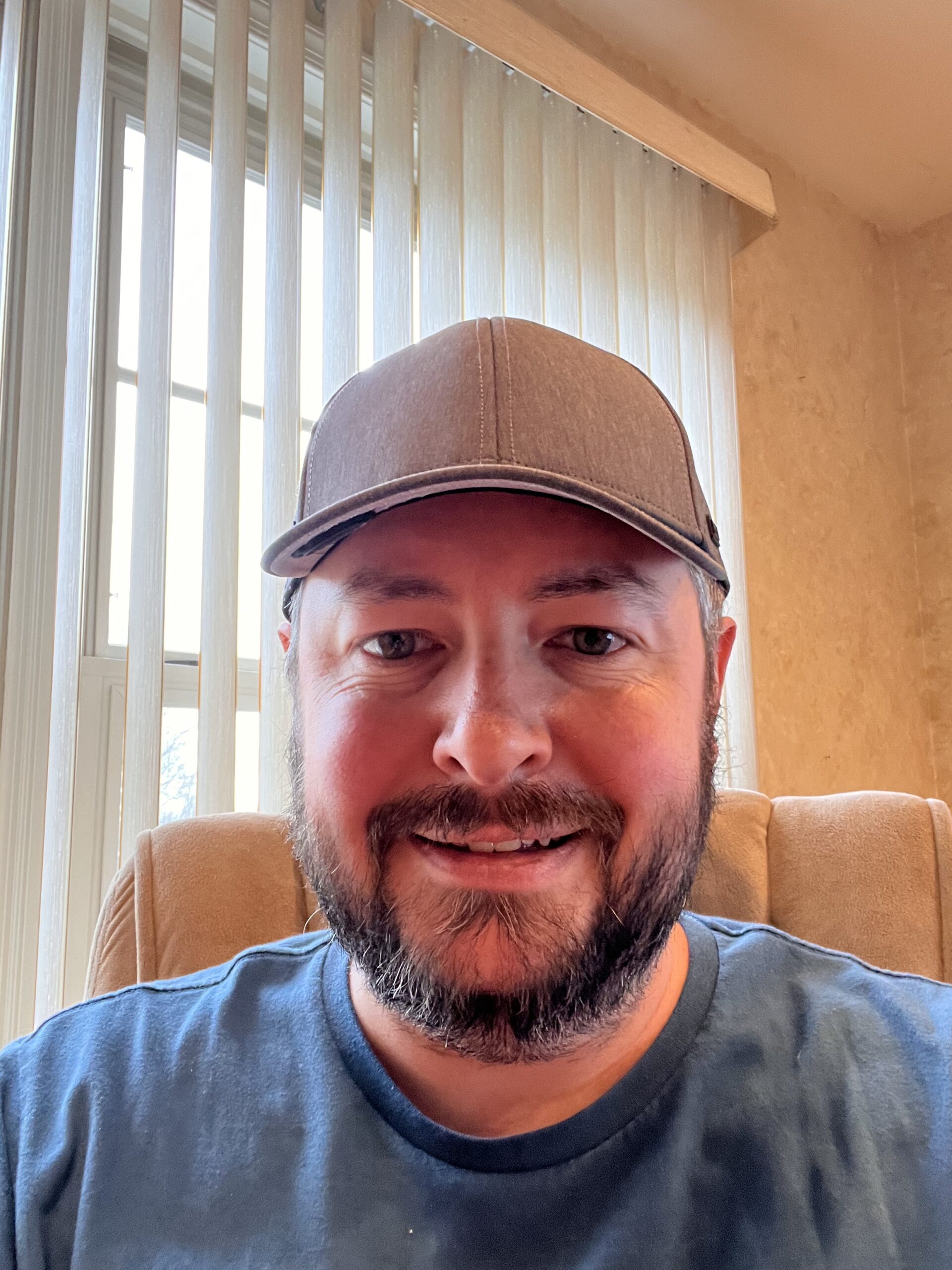
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.