Have you ever been happily coding away in Python when suddenly you’re hit with a TypeError that says something about a ‘type’ object? Don’t worry, you’re not alone. This error can be a bit confusing, but once you understand what it means, it’s usually pretty easy to fix. In this article, we’ll go through what a ‘type’ object issue is, how to troubleshoot it step by step, and some tips to help you avoid it in the future.
Understanding TypeError in Python: ‘type’ Object Issues
Before we dive into the steps to fix a ‘type’ object issue, let’s briefly talk about what this error means. In Python, everything is an object, including the types themselves. When you see a TypeError that mentions a ‘type’ object, it means that you’re trying to perform an operation or use a method that isn’t supported by that particular type.
Step 1: Identify the Line of Code Causing the Error
The first thing you need to do is figure out which line of code is causing the TypeError. Python will usually give you a traceback that includes the file name, line number, and the code on that line.
Once you’ve found the line of code, take a closer look at the operation or method being used. Is it something that should work with the type you’re using?
Step 2: Check the Type of the Object
If the operation should work with the type, the next step is to check the type of the object you’re trying to use it on. You can do this by using the built-in type() function.
Sometimes, the object may not be the type you expected, which is why the operation is failing. For example, you may be trying to concatenate a string with an integer, which will result in a TypeError.
Step 3: Fix the Type Mismatch
Once you’ve identified the type mismatch, you can fix the error by either converting the object to the correct type or by using a different operation or method that is supported by the type.
For example, if you’re trying to concatenate a string with an integer, you can convert the integer to a string using the str() function before concatenating.
After you’ve fixed the type mismatch, the TypeError should be resolved, and your code should run without any issues.
Tips for Avoiding ‘type’ Object Issues in Python
- Always be aware of the type of the objects you’re working with.
- Use type annotations to specify the expected type of function arguments and return values.
- Use type checking tools like MyPy to catch type errors before running your code.
- Be cautious when using dynamic typing, as it can lead to unexpected type mismatches.
- Write unit tests that include type-related edge cases to ensure your code can handle them.
Frequently Asked Questions
What is a ‘type’ object in Python?
A ‘type’ object in Python represents the type of an object. It’s used to define the operations that the object supports and the attributes it contains.
Can I create my own ‘type’ object?
Yes, you can create your own ‘type’ object by defining a new class. The class will then become a new type in Python.
What does the TypeError message "object is not callable" mean?
This message means that you’re trying to call an object as if it were a function, but the object doesn’t support being called.
How do I check the type of an object in Python?
You can check the type of an object by using the built-in type() function, which will return the ‘type’ object of the given object.
Can type errors be prevented at compile time in Python?
Python is a dynamically typed language, which means that types are checked at runtime, not compile time. However, you can use type annotations and type checking tools to catch type errors before running your code.
Summary
- Identify the line of code causing the TypeError.
- Check the type of the object involved.
- Fix the type mismatch by converting the object or using a different operation.
Conclusion
Understanding the ‘type’ object issues in Python can be perplexing at first, but with a little bit of knowledge and the right approach, they’re not so scary. Remember to always keep track of the types you’re working with, and don’t hesitate to use the type() function to check if you’re unsure. By following the steps outlined in this article, you’ll be able to quickly diagnose and fix any TypeError that comes your way. Happy coding, and may your Python journey be free from ‘type’ object issues!
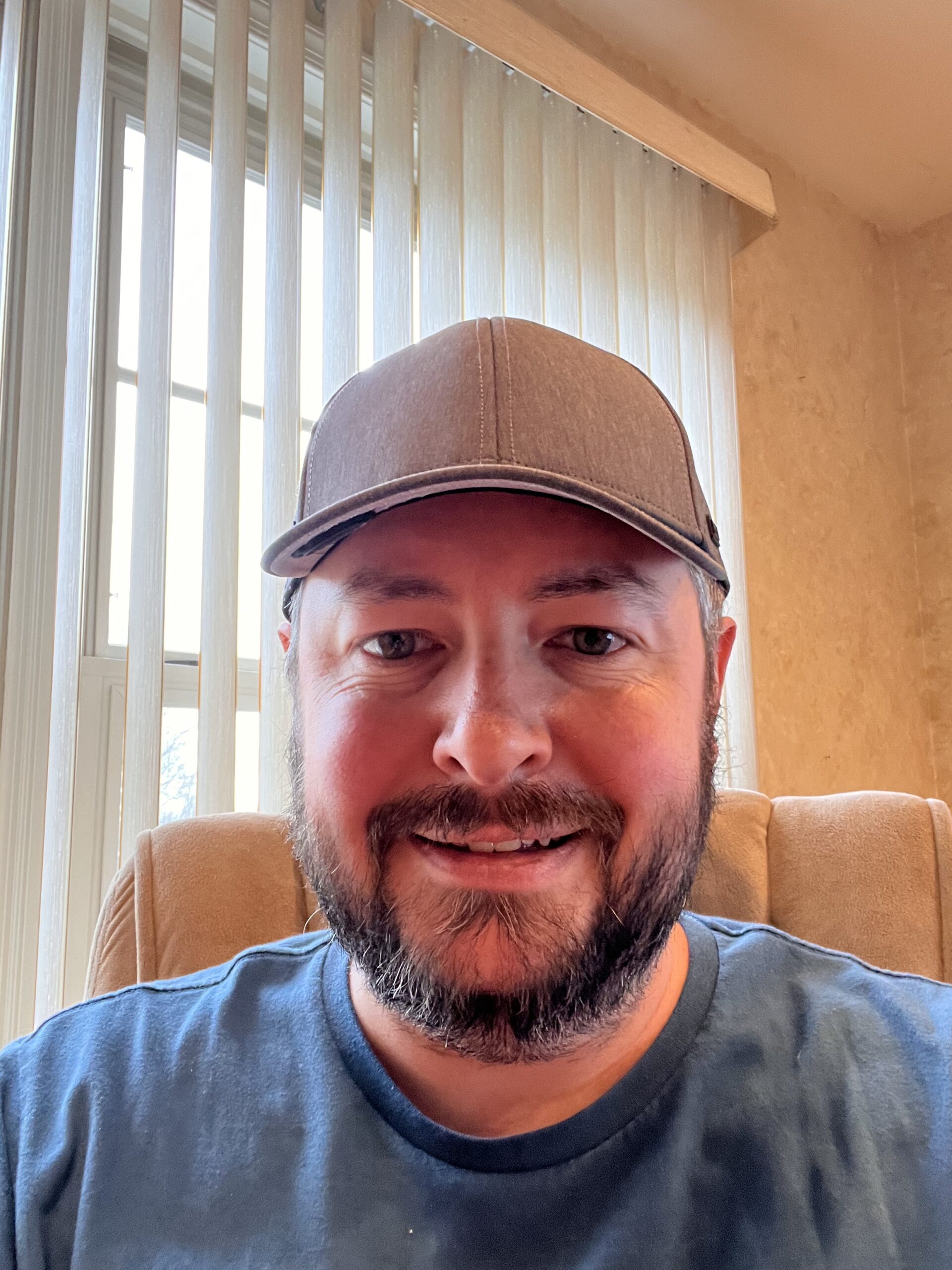
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.