Fixing the ‘Index Out of Range’ error in Python list assignments may seem daunting, but it’s quite simple once you understand the basics. This error occurs when you try to access an index in a list that doesn’t exist. By following a few steps, you can easily correct your code and avoid this common mistake.
Step by Step Tutorial: Fixing Python List Assignments
Before diving into the steps, it’s important to understand that lists in Python are zero-indexed. This means that the first element is at index 0, the second element at index 1, and so on. The ‘Index Out of Range’ error happens when you attempt to access an index that is higher than the last index in your list.
Step 1: Identify the Problematic Index
The first step is to figure out which index is causing the error.
Oftentimes, the error message will tell you exactly which line of code is problematic. Look for the index that is being accessed and compare it with the length of your list. If the index is equal to or larger than the length of your list, that’s your issue.
Step 2: Modify the Index or List
Once you’ve identified the problematic index, you need to either adjust the index or modify the list itself.
If the index is a hardcoded number, consider whether it’s necessary to access that specific index, or if it was a mistake. If the index is a result of a calculation or loop, check the logic to ensure it stays within the bounds of the list. Alternatively, you may need to expand your list by appending additional elements to avoid the error.
Step 3: Test Your Solution
After making the necessary changes, run your code again to see if the error persists.
Testing is crucial because it ensures that your solution works as intended. If the ‘Index Out of Range’ error is gone, congratulations! If not, revisit the previous steps and double-check your logic and list manipulations.
Once you’ve completed these steps, your Python list assignments should be error-free, and you can continue coding without the pesky ‘Index Out of Range’ error.
Tips: Avoiding ‘Index Out of Range’ Errors
- Always check the length of your list before accessing an index.
- Use loops carefully, ensuring that the iteration doesn’t exceed the list’s length.
- Consider using list methods like append() or extend() to dynamically adjust list sizes.
- Utilize exception handling with try and except blocks to catch and handle errors gracefully.
- Familiarize yourself with Python’s list slicing to access ranges of elements safely.
Frequently Asked Questions
What does ‘Index Out of Range’ mean?
It means that you’re trying to access an element in a list using an index number that doesn’t exist. Since lists are zero-indexed, the last index will always be one less than the length of the list.
Can I use negative indices in Python lists?
Yes, Python allows the use of negative indices to access elements from the end of a list. For example, -1 refers to the last element, -2 to the second to last, and so on.
How do I avoid ‘Index Out of Range’ errors in loops?
Make sure that your loop condition or range doesn’t exceed the list’s length. For example, use for i in range(len(my_list)): to ensure the loop stays within bounds.
What is the best practice for accessing the last element of a list?
You can use the index -1 to access the last element of a list, as it’s more readable and doesn’t require knowing the exact length of the list.
Should I always check the list length before accessing an element by index?
While it’s good practice to be cautious, if you’re certain of the list’s size or the index is well within the expected range, it may not be necessary. However, always validate indices when dealing with dynamic or unknown list sizes.
Summary
- Identify the problematic index causing the ‘Index Out of Range’ error.
- Modify the index or list to ensure the index exists within the list.
- Test your code to confirm the error is resolved.
Conclusion
Fixing the ‘Index Out of Range’ error in Python list assignments is a skill that every Python programmer should master. It involves understanding how list indexing works, carefully managing list lengths, and being mindful of looping constructs. Remember, the key to avoiding this error is to ensure that any index you try to access is within the bounds of your list. Keep practicing, and soon, dealing with list assignments and indexes will be second nature. If you ever get stuck, revisit the steps in this article, apply the tips, and consult the frequently asked questions for guidance. Happy coding!
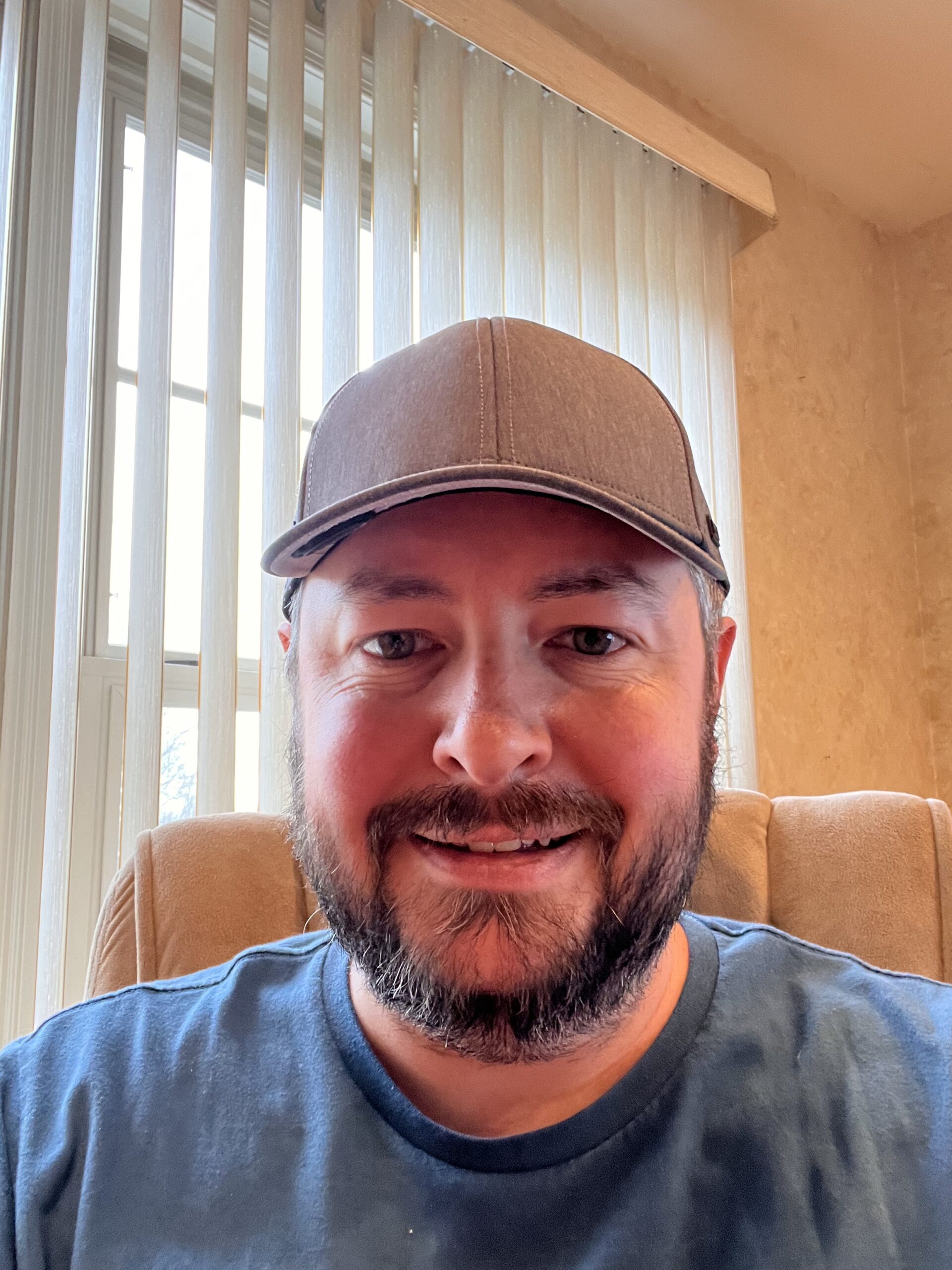
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.