In programming, it’s crucial to make sure that the input data you’re using falls within a specified range. This helps prevent errors and ensures that your program runs smoothly. But how do you do that? Well, it’s not as complicated as it sounds. With a few simple steps, you can set up your program to check the data and make sure it’s just right.
Step by Step Tutorial: Ensuring Input Data Falls Within a Specified Range
Before we dive into the steps, it’s important to understand why we’re doing this. Checking that your input data is within a specified range helps prevent unexpected behavior in your program. It’s a way to catch errors early on and fix them before they cause bigger problems. Let’s get started.
Step 1: Define the Range
Start by defining the range of acceptable values for your input data.
Defining the range of acceptable values is the first step in making sure your input data is valid. This could be a range of numbers, such as 1-10, or a set of specific values, like "yes," "no," or "maybe." By setting these parameters, you’re creating a rule for your program to follow.
Step 2: Validate the Input
Next, write a function that checks the input data against the defined range.
Validating the input means comparing it to the range you’ve defined. If the input data falls within the range, your program can proceed as normal. If it doesn’t, the function should trigger an error message or prompt the user to enter a new value. This step is crucial for maintaining data integrity.
Step 3: Handle Invalid Input
Decide how your program will handle data that doesn’t fall within the specified range.
Handling invalid input might mean prompting the user to re-enter the data, or it could mean terminating the program with an error message. Whatever approach you choose, it should be consistent and clear to the user. This step ensures that your program doesn’t continue with bad data.
Step 4: Test Your Code
Finally, test your code with a variety of input values to ensure it’s working as expected.
Testing your code with different inputs is like a practice run for your program. Try values that are within the range, on the edge of the range, and outside of the range. This will help you catch any bugs or issues before the program is used in a real-world situation.
After completing these steps, your program should be equipped to handle input data more reliably. It will check the data against the specified range, and if it doesn’t fit, the program will know exactly what to do. This adds an extra layer of security and stability to your code.
Tips: Ensuring Input Data Falls Within a Specified Range
- Always define the range before writing the validation function. It’s your blueprint for what’s acceptable.
- Use clear error messages that help the user understand what went wrong.
- Consider edge cases when defining your range, like zero or negative numbers.
- Make sure to test your code thoroughly with a variety of inputs.
- Keep your validation function separate from other parts of your code for easier maintenance.
Frequently Asked Questions
What happens if I don’t check the input data range?
If you don’t check the input data range, your program might behave unpredictably or crash when it receives unexpected data. It’s like trying to fit a square peg in a round hole – something’s going to go wrong.
Can I use this method with any programming language?
Yes, the concept of checking input data against a specified range is universal in programming. The specific syntax will vary depending on the language, but the steps are the same.
What should I do if I’m not sure what range to use?
If you’re unsure about the range, consider the context of the data. What makes sense for the program you’re writing? If you’re still stuck, do some research or ask a fellow programmer for advice.
How can I make sure my validation function is efficient?
To make your validation function efficient, keep it simple. The less complex it is, the quicker it will run. Also, avoid unnecessary calculations or comparisons.
Can I have multiple ranges for different types of input data?
Absolutely! You can define as many ranges as you need for different types of input data. Just make sure each range is clearly defined and that your validation function checks each one appropriately.
Summary
- Define the range of acceptable values.
- Write a function to check the input against the range.
- Decide how to handle data outside the range.
- Test your code with various input values.
Conclusion
Ensuring input data falls within a specified range is a fundamental aspect of programming that can save you a lot of headaches down the line. It’s like setting the rules of the game before you start playing. By following the steps we’ve outlined, you can create a program that’s robust, reliable, and ready for anything users throw at it. And remember, testing is your best friend. The more you test, the more confident you can be that your program will perform as expected. So, roll up your sleeves, define those ranges, and start coding with confidence. Happy programming!
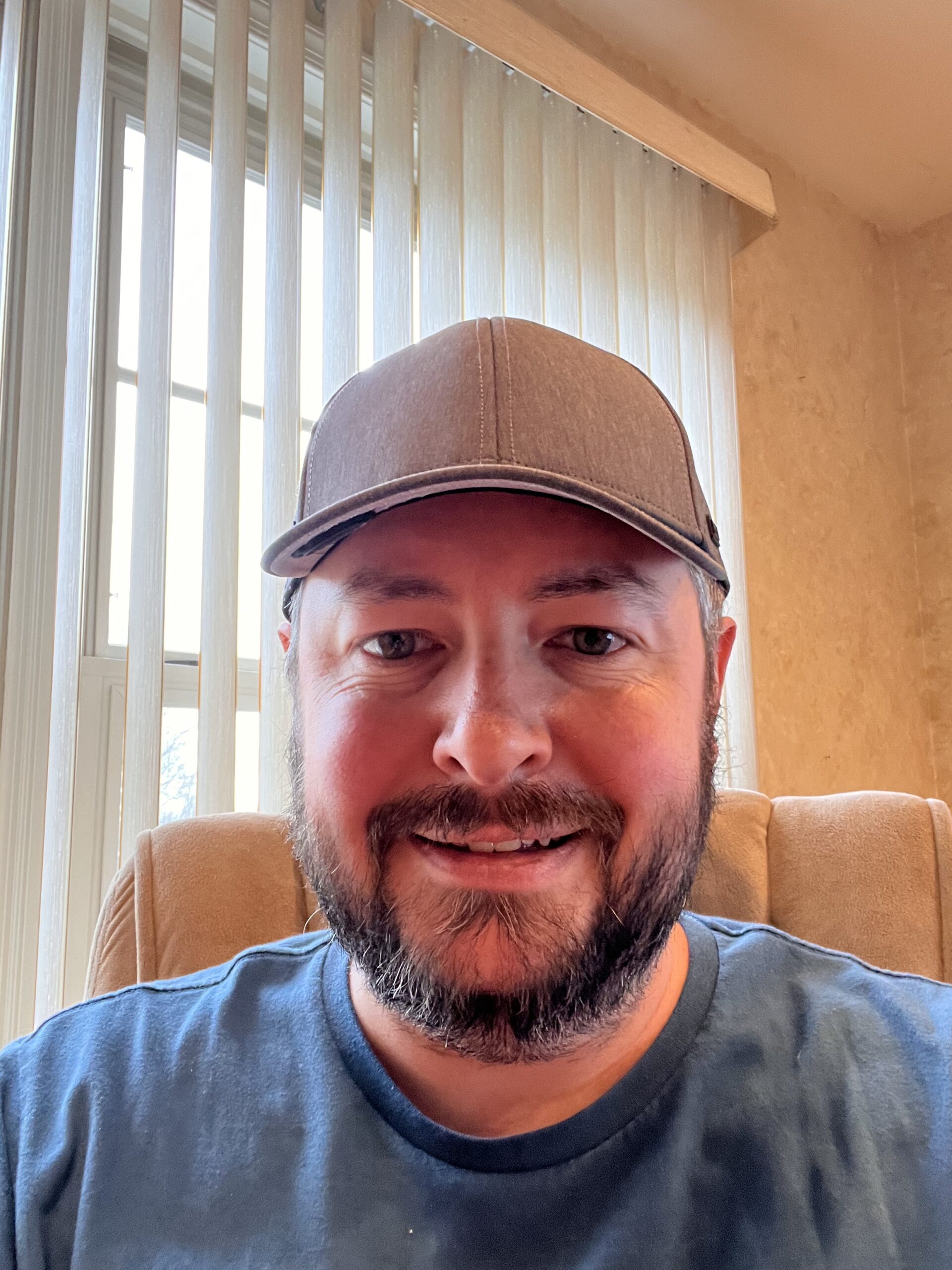
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.