Formatting strings in Python can seem daunting at first, but once you get the hang of it, it’s a breeze! If you’ve ever encountered a ‘TypeError’ issue while working with strings, it’s probably because you’re trying to combine a string with a non-string type, like an integer or a float. To fix this, you’ll need to convert the non-string type into a string before you can concatenate it with other strings. Let’s dive into the steps you need to take to format strings in Python and avoid those pesky ‘TypeError’ issues.
Step by Step Tutorial: Formatting Strings in Python
Before we start fixing ‘TypeError’ issues, it’s important to understand that strings in Python are sequences of characters that are enclosed in quotes. You can use either single or double quotes. Now, let’s go through the steps to format strings properly.
Step 1: Identify the ‘TypeError’
The first thing you need to do is identify where the ‘TypeError’ is occurring in your code. This error usually happens when you’re trying to concatenate different types, like a string and an integer.
When you find the line of code that’s causing the error, take a close look at what you’re trying to concatenate. If you see something like print("The number is " + 42)
, you’ll know that’s where the problem is because you’re trying to add a string and an integer together.
Step 2: Convert Non-String Types to String
To fix the ‘TypeError’, you’ll need to convert any non-string types to strings before concatenating them. You can do this using the str()
function in Python.
If you’re fixing the error from step 1, you would change the code to print("The number is " + str(42))
. This converts the integer 42 to a string, allowing you to concatenate it with other strings without any issues.
Step 3: Use String Formatting Methods
Python provides several methods for string formatting that can make your life easier. Two popular methods are the format()
method and f-strings (formatted string literals).
Using the format()
method, you can rewrite our previous example as print("The number is {}".format(42))
. If you’re using Python 3.6 or later, you can use an f-string like this: print(f"The number is {42}")
. Both of these methods automatically handle the conversion of non-string types to strings.
Step 4: Test Your Code
After making the necessary changes, run your code again to make sure the ‘TypeError’ is gone. This will confirm that you’ve formatted the strings correctly.
If your code runs without any errors, congratulations! You’ve successfully formatted your strings and resolved the ‘TypeError’ issue.
After completing these steps, you should have code that runs smoothly without any ‘TypeError’ issues. You’ll now be able to combine strings with other data types without any hiccups.
Tips: Avoiding ‘TypeError’ Issues in Python
- Always use the
str()
function to convert non-string types to strings before concatenating. - Familiarize yourself with Python’s string formatting methods like
format()
and f-strings. - Pay close attention to the data types you’re working with when dealing with strings.
- Test your code regularly to catch errors early.
- Read error messages carefully; they often tell you exactly where and what the issue is.
Frequently Asked Questions
What is a ‘TypeError’ in Python?
A ‘TypeError’ occurs when you try to perform an operation on a value that is not of the expected type, such as trying to concatenate a string with an integer.
How do I concatenate strings in Python?
You can concatenate strings in Python using the +
operator, as long as all the elements are strings. If not, you’ll need to convert the non-string elements using the str()
function.
What is the str()
function in Python?
The str()
function in Python is used to convert a given value into a string. It’s especially useful for converting non-string types like integers and floats when you need to concatenate them with strings.
What are f-strings in Python?
F-strings, introduced in Python 3.6, are a way to format strings that is both concise and easy to read. They allow you to include expressions inside string literals using curly braces {}
.
Can I use f-strings in older versions of Python?
No, f-strings are only available in Python 3.6 and later. If you’re using an older version, you can use the format()
method instead.
Summary
- Identify the ‘TypeError’.
- Convert non-string types to strings using
str()
. - Use string formatting methods like
format()
or f-strings. - Test your code.
Conclusion
Dealing with ‘TypeError’ issues while formatting strings in Python can be frustrating, but with the right approach, it’s a problem that can be easily solved. By identifying where the error occurs, converting non-string types to strings, and making use of Python’s string formatting methods, you can ensure your code is clean and error-free. Remember to test your code after making changes to confirm that the issue is resolved. With these tips and steps in mind, you should be well on your way to becoming a pro at formatting strings in Python. Keep practicing, and don’t be afraid to experiment with different methods to find what works best for you. Happy coding!
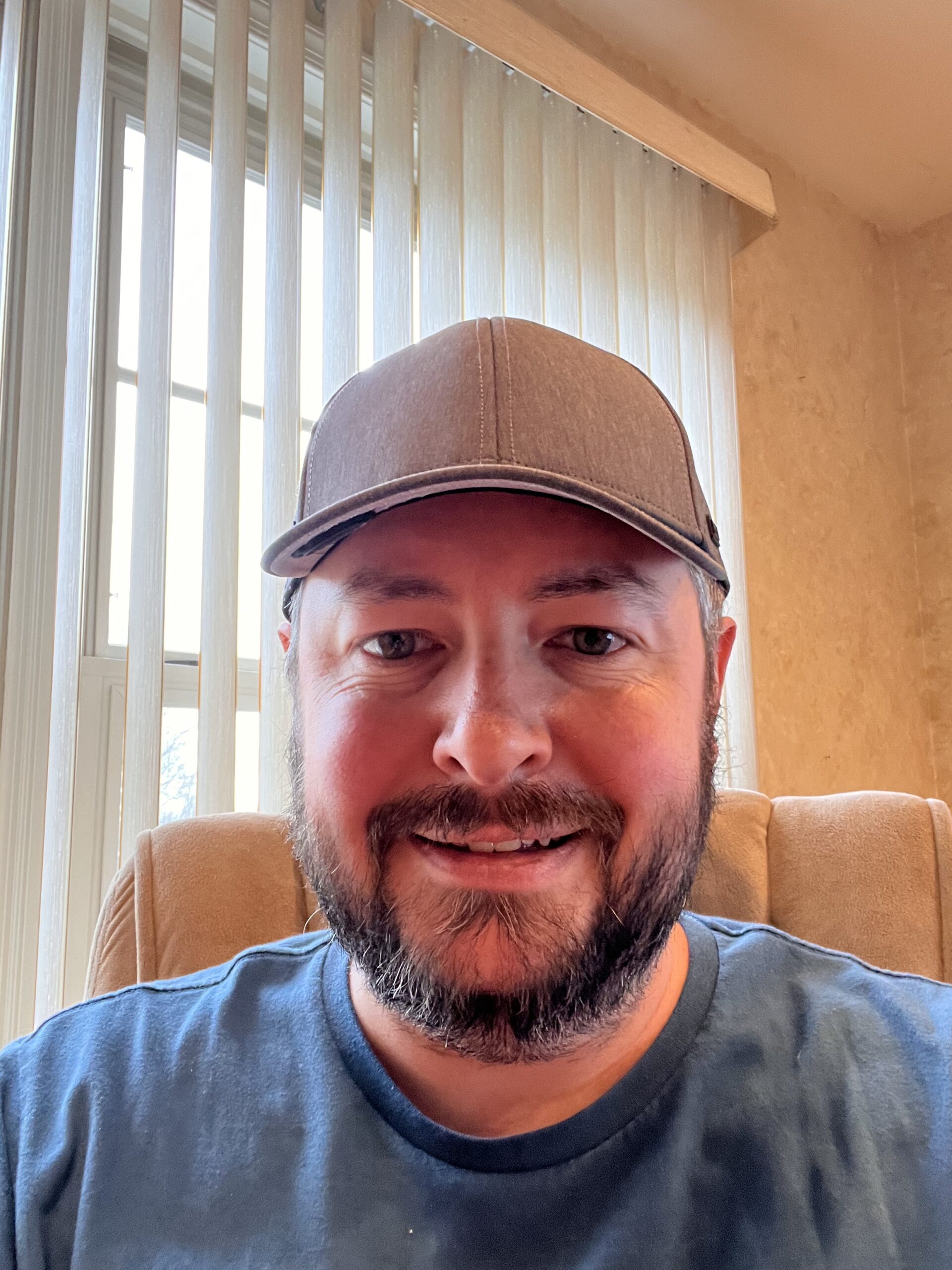
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.