Regex, or regular expressions, can be a programmer’s best friend or worst enemy. They are powerful tools for pattern matching in strings of text, but when they don’t work as expected, it can be a real headache. Resolving regex pattern mismatches requires a keen eye for detail and a solid understanding of regex syntax. In this article, we’ll walk through the steps to troubleshoot and fix these mismatches, ensuring your code runs smoothly.
Step by Step Tutorial: Resolving Regex Pattern Mismatches
Before we dive into the nitty-gritty, let’s understand what the following steps will achieve. This tutorial will guide you through identifying the regex pattern mismatch, testing your regex, and implementing the fix to get your code back on track.
Step 1: Identify the Mismatch
Identify the specific pattern that is causing the mismatch in your code.
When a regex pattern mismatch occurs, it means that the pattern you’ve written isn’t matching the strings you expect it to. To fix this, you need to pinpoint the exact pattern that’s causing the problem. Look at the results you’re getting and compare them to the results you want. This will help you understand where the discrepancy lies.
Step 2: Understand the Regex Syntax
Ensure you have a clear understanding of regex syntax and the rules governing the pattern you’re using.
Regex syntax can be quite complex, with various characters and sequences that perform different matching functions. If you’re encountering mismatches, it’s a good idea to refresh your knowledge of regex syntax to make sure you’re using the right pattern for the job.
Step 3: Test Your Regex
Use a regex testing tool to experiment with your pattern and see where it fails.
There are many online tools available that allow you to test regex patterns against sample text. These tools often highlight which parts of the sample text are matched by your pattern, making it easier to see where the pattern is failing and why.
Step 4: Adjust Your Pattern
Modify your regex pattern to correct the mismatch and test it again.
Once you’ve identified where the mismatch is occurring, adjust your pattern to match the strings you want. This may involve adding or removing characters, changing quantifiers, or incorporating different regex constructs. Test your adjusted pattern with the same tool to ensure it’s now working as expected.
Step 5: Implement the Fix
Apply the corrected regex pattern to your code and verify that the mismatch is resolved.
After testing and confirming that your adjusted pattern is working correctly, it’s time to implement the fix in your code. Replace the old pattern with the new one and run your program to ensure that the mismatch has been resolved.
After completing these steps, your regex pattern should be matching the correct strings, and any mismatches should be resolved. This means you can move on with your programming tasks without the frustration of unexpected results.
Tips for Resolving Regex Pattern Mismatches
Here are some useful tips to keep in mind when working with regex patterns:
- Always test your patterns with a variety of sample strings to ensure they’re robust.
- Remember that regex can behave differently in different programming languages or environments, so check the documentation specific to your context.
- Keep your patterns as simple as possible. Overly complex patterns can be harder to debug.
- Use comments to explain particularly complex regex patterns for future reference.
- Consider using regex libraries or modules that offer additional functionality or simplified syntax.
Frequently Asked Questions
What is a regex pattern mismatch?
A regex pattern mismatch occurs when a regex pattern doesn’t match the strings that it’s supposed to match.
Regex is used for pattern matching, and sometimes, due to incorrect syntax or logic, the pattern you’ve written doesn’t catch the strings you’re targeting. When this happens, it’s called a regex pattern mismatch.
How do I know if my regex pattern has a mismatch?
You’ll know there’s a mismatch if your pattern is not capturing the strings you expect, or if it’s capturing strings it shouldn’t.
When testing your code, if the output isn’t what you anticipated based on your regex pattern, that’s a clear sign of a mismatch. Using regex testing tools can also help you identify these issues.
Can regex patterns be different in different programming languages?
Yes, regex implementations can vary between programming languages, so patterns may not work the same across all of them.
While the core concepts of regex are relatively consistent, the specific syntax and available features can differ between languages. Always refer to the documentation for the language you’re using.
Are there tools to help me test my regex patterns?
Absolutely, there are many online regex testing tools that can help you visualize and debug your patterns.
These tools allow you to enter your regex pattern and test strings, and then they show you exactly which parts of the test strings are matched. This immediate feedback can be incredibly helpful for resolving mismatches.
Can I use comments in my regex patterns?
In some regex engines, yes, you can use comments to clarify complex patterns, but it depends on the implementation.
Some regex engines support a mode where whitespace and comments are ignored within the pattern, allowing for more readable and maintainable regex. Check if this feature is available in the language or tool you’re using.
Summary
- Identify the specific pattern causing the mismatch.
- Refresh your knowledge of regex syntax.
- Test your regex with an online tool.
- Adjust the pattern to correct the mismatch.
- Implement the corrected pattern in your code.
Conclusion
Regex patterns are a fundamental tool in a programmer’s toolkit, but they can also be a source of frustration when they don’t work as expected. Resolving regex pattern mismatches requires a systematic approach to identify, test, and correct the pattern. By following the steps outlined in this article, you should be able to tackle any regex issues that come your way confidently. Remember to use online testing tools to your advantage, keep your patterns simple, and always refer to the documentation specific to your programming environment. With practice and patience, you’ll become a regex pro in no time, and pattern mismatches will be a thing of the past. Happy coding!
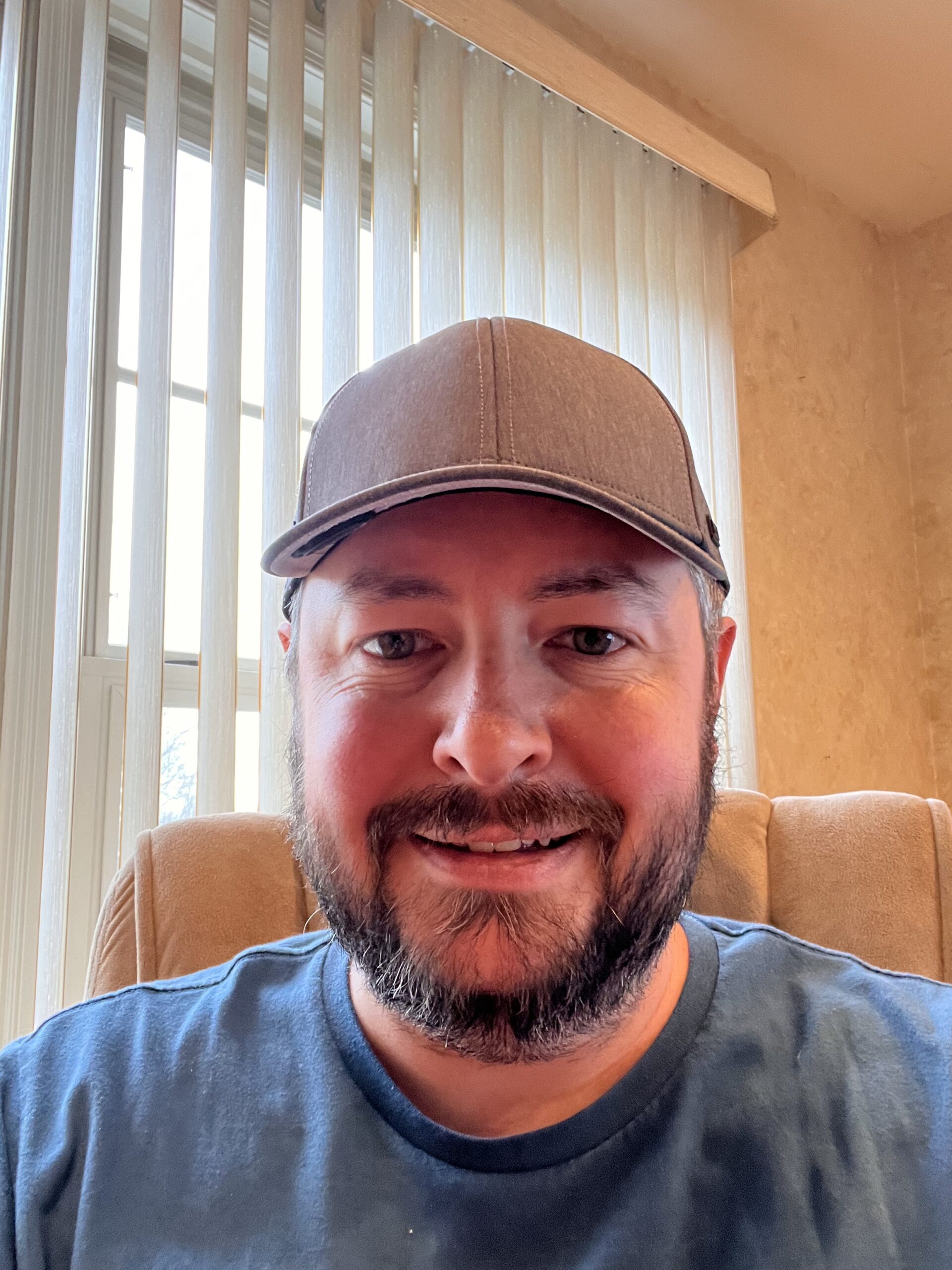
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.