Converting strings to dictionaries in Python can be an incredibly useful skill when working with data. It allows you to take a string that represents key-value pairs and turn it into a dictionary, which is a more organized and versatile data structure. This can be especially helpful when dealing with JSON data or when you need to manipulate data in a more structured way.
Step by Step Tutorial: Converting Strings to Dictionaries in Python
Before diving into the steps, it’s important to understand what we’re aiming to achieve. Converting a string to a dictionary in Python allows us to take a piece of data that is formatted as a string and transform it into a dictionary. A dictionary in Python is a collection of key-value pairs, which makes it easier to work with and manipulate the data.
Step 1: Import the json module
To start, you’ll need to import the json module, which will help us convert the string to a dictionary.
The json module is a part of Python’s standard library and is specifically designed to work with JSON data. It provides methods for parsing JSON strings and converting them into Python objects, such as dictionaries.
Step 2: Create a string that you want to convert
Next, you’ll need to create a string that represents the data you want to convert into a dictionary.
The string should be in a format that can be easily converted to a dictionary, typically a JSON format. This means it should contain key-value pairs enclosed in curly braces, with keys and values separated by colons and pairs separated by commas.
Step 3: Use json.loads() to convert the string to a dictionary
Now, you can use the json.loads() method to convert the string into a dictionary.
The json.loads() method takes a string as input and returns a dictionary. It parses the string, identifies the key-value pairs, and creates a dictionary with the corresponding structure.
Step 4: Print the dictionary to confirm the conversion
Finally, print out the dictionary to confirm that the conversion has been successful.
After converting the string to a dictionary, you can print it out to see the result. The dictionary should contain all the key-value pairs from the original string in a structured and easily accessible format.
After completing these steps, you will have successfully converted a string to a dictionary in Python. This dictionary can then be used for various purposes, such as data manipulation, analysis, or even for passing as an argument to a function.
Tips: Converting Strings to Dictionaries in Python
- Ensure that the string is in a proper JSON format before attempting to convert it into a dictionary.
- If the string contains single quotes, make sure to replace them with double quotes, as JSON requires double quotes.
- Use the try-except block to handle any errors that may occur during conversion, such as JSONDecodeError.
- Remember that keys in a Python dictionary must be unique, so ensure that the string doesn’t contain duplicate keys.
- After conversion, you can use all the standard dictionary methods to manipulate the data, such as accessing values by keys, updating values, or adding new key-value pairs.
Frequently Asked Questions
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is widely used for transmitting data in web applications.
Can I convert a string to a dictionary without the json module?
Yes, you can manually parse the string and construct the dictionary, but it is more error-prone and not recommended when the json module provides a simple and reliable method.
What if my string is not in JSON format?
If your string is not in JSON format, you’ll need to modify it to match the expected format or use a different method to parse and convert the string.
How do I handle nested dictionaries or lists within the string?
The json.loads() method can handle nested dictionaries and lists within the string, and it will convert them into corresponding Python data structures.
Can I convert a dictionary back into a string?
Yes, you can use the json.dumps() method to convert a dictionary back into a JSON-formatted string.
Summary
- Import the json module.
- Create a string in JSON format.
- Use json.loads() to convert the string to a dictionary.
- Print the dictionary to confirm the conversion.
Conclusion
Converting strings to dictionaries in Python is a valuable skill that can significantly improve the way you handle and manipulate data. By following the simple steps outlined in this article, you can quickly and easily transform a string into a more structured and useful dictionary. This process is crucial for working with JSON data, which is common in web development and data analysis. Remember to ensure that the string is in the proper format and to handle any potential errors that may arise during conversion. With these tips and techniques, you’ll be well-equipped to work with data more efficiently and effectively in Python.
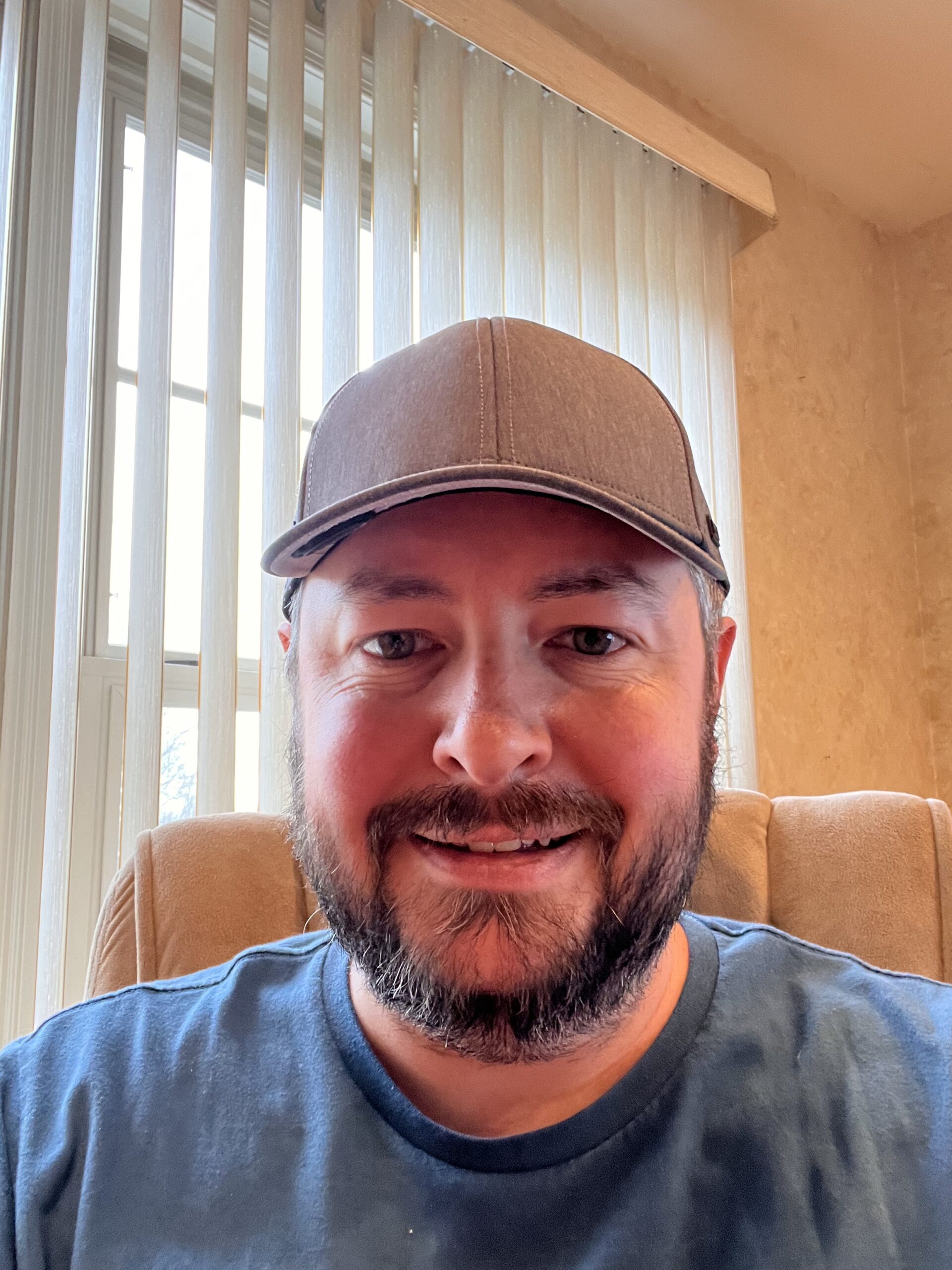
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.