How to Split a Word in Python
Splitting a word in Python is super simple and handy, especially when you want to break down strings into smaller parts. By using Python’s built-in string methods, you can easily split words based on spaces, specific characters, or even fixed lengths. This article will walk you through the steps to split a word, with a detailed tutorial and helpful tips to make the process smoother.
Splitting a Word in Python
In this tutorial, you’ll learn how to split a word in Python using the split() method and some other techniques. Let’s get started so you can master this essential skill.
Step 1: Understand the split() Method
The split() method divides a string into a list based on a specified separator.
This method is like cutting a cake; it lets you decide where to make the slices. If you don’t specify a separator, it defaults to splitting by spaces.
Step 2: Use split() with a Space Separator
To split a word into individual characters, you can use an empty string as the separator.
For example, word = "hello"
, doing list(word)
will split it into ['h', 'e', 'l', 'l', 'o']
. This directly converts the word into a list of its characters.
Step 3: Split by Specific Character
You can also specify a separator to split by a particular character.
For instance, if you have word = "h-e-l-l-o"
and you use word.split('-')
, you’ll get ['h', 'e', 'l', 'l', 'o']
. This is useful for words or strings separated by dashes, commas, etc.
Step 4: Splitting Using Fixed Lengths
Sometimes you might need to split a string into chunks of a fixed length.
This can be done using a loop or list comprehension, like so: [word[i:i+2] for i in range(0, len(word), 2)]
for a length of 2. This will split word = "hello"
into ['he', 'll', 'o']
.
Step 5: Using re.split() for Advanced Splitting
For more complex splitting, using regular expressions with the re module is powerful.
Import the re module with import re
, and then use re.split(pattern, word)
. For example, re.split(r'[aeiou]', 'hello')
will split the word at every vowel, resulting in ['h', 'll', '']
.
After completing these steps, you will have successfully split a word in Python using different methods. You can now handle various scenarios efficiently.
Tips for Splitting a Word in Python
- Experiment with different separators: Try splitting with different characters like commas, spaces, or even special characters.
- Always check the output: Ensure the list produced matches your expectations.
- Use list comprehensions: They are concise and powerful for more complex splits.
- Handle edge cases: Test with empty strings or strings with no separators.
- Practice with real data: Try splitting words from a text file to see how these methods work in real-world scenarios.
Frequently Asked Questions
What does the split() method return?
The split() method returns a list of strings.
This list contains the parts of the original string divided based on the specified separator.
Can I split a word without spaces?
Yes, you can split a word without spaces by using an empty string as a separator.
For example, using list(word)
directly converts the word into a list of its characters.
How do I split using multiple characters as separators?
You can use the re module to split using multiple characters.
For example, re.split(r'[,-]', 'h,e-l,l-o')
will split the word at both commas and dashes.
What happens if no separator is specified in split()?
If no separator is specified, split() defaults to splitting by spaces.
This is useful for breaking down sentences into words.
How to split a string into fixed-length chunks?
You can use a loop or list comprehension to split a string into fixed-length chunks.
For instance, [word[i:i+2] for i in range(0, len(word), 2)]
will split a string into chunks of length 2.
Summary
- Understand the split() method.
- Use split() with a space separator.
- Split by specific character.
- Splitting using fixed lengths.
- Using re.split() for advanced splitting.
Conclusion
Splitting a word in Python is a fundamental skill that can make your text processing tasks a breeze. Whether you’re breaking down simple strings or handling more complex text manipulations, knowing how to use Python’s built-in methods like split() and the re module will save you time and effort.
Don’t forget to experiment with different separators and test with real data to see how these methods can be applied in various scenarios. This guide should get you started on the right foot, but there’s always more to learn. Keep practicing, and soon you’ll be splitting words in Python like a pro. For more in-depth tutorials on Python string handling, check out some additional resources and keep enhancing your coding skills!
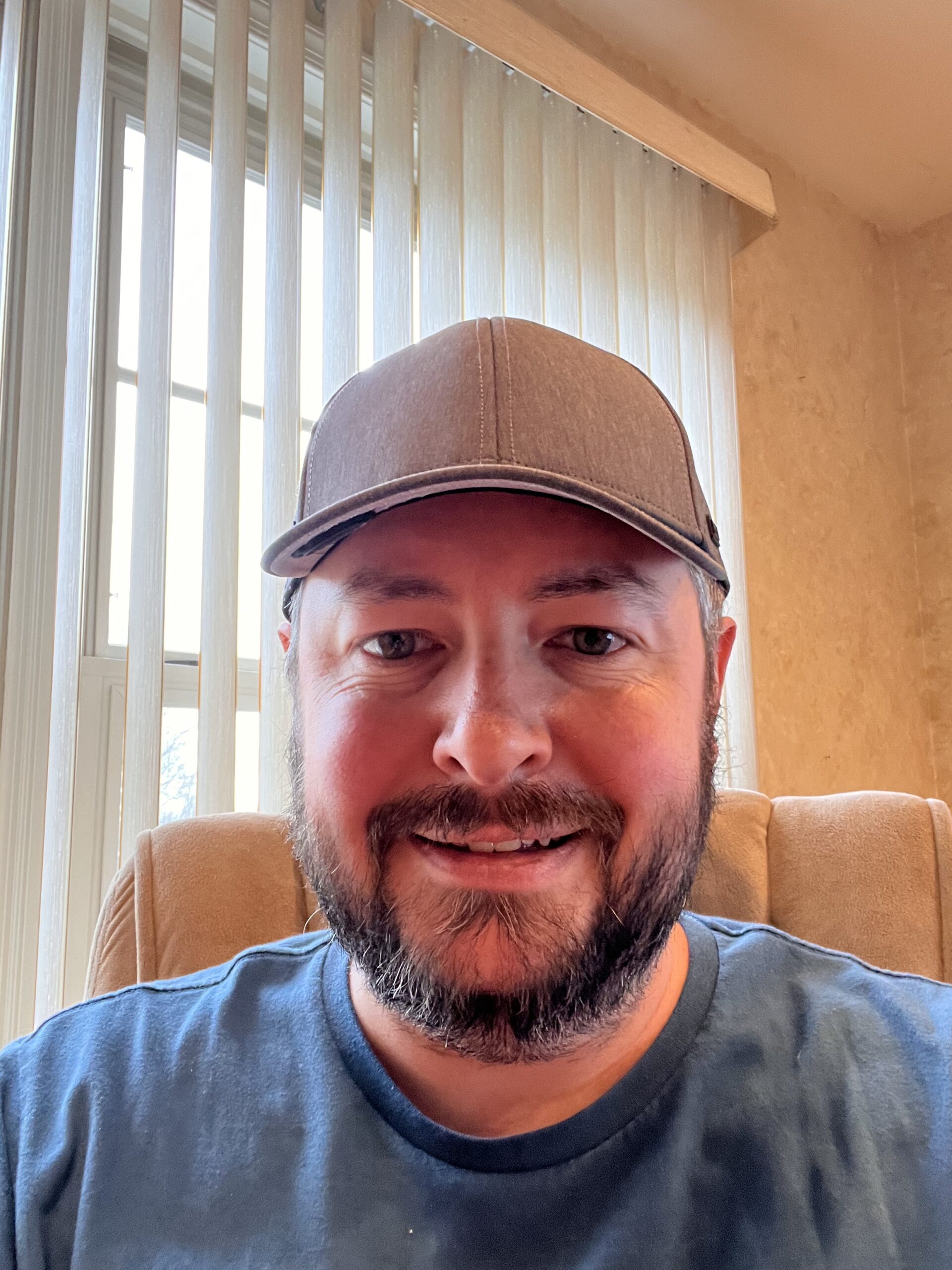
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.