Replacing a word in Python is a breeze! You can use the built-in string methods to effortlessly swap out one word for another within a string. This guide will walk you through the process step-by-step, ensuring you can confidently replace words in your Python scripts in no time.
How to Replace a Word in Python
In this section, we will demonstrate how to replace a word in a string using Python. By following these simple steps, you will learn how to use Python’s string manipulation methods to change words within text.
Step 1: Open your Python environment
Open your preferred Python development environment, such as IDLE, Jupyter Notebook, or any text editor that supports Python.
You need an environment where you can write and execute Python code. If you don’t have one, download and install Python from the official website.
Step 2: Define your string
Create a variable and assign your string to it. For example, my_string = "Hello world!"
.
This string will be the text where you want to replace a word. Ensure it contains the word you want to change.
Step 3: Use the replace() method
The replace() method is used to replace a word. For example, my_string.replace("world", "Python")
.
The replace() method takes two arguments: the word you want to replace, and the word you want to replace it with. This method will return a new string with the specified replacement.
Step 4: Save the new string
Assign the result of the replace() method to a new variable or overwrite the existing variable, like new_string = my_string.replace("world", "Python")
.
This step ensures that you store the modified string for further use. If you don’t assign it, the original string remains unchanged.
Step 5: Print the new string
Print the new string to see the changes: print(new_string)
.
Printing the string will show you if the word replacement was successful. It verifies that your code is working as expected.
After completing these steps, your original string will have the specified word replaced with the new word. The replace() method is effective and user-friendly, making word replacement in Python straightforward.
Tips for Replacing a Word in Python
- Double-check the word you want to replace to ensure it’s correctly specified.
- The replace() method is case-sensitive, so "world" and "World" are treated as different words.
- You can chain replace() methods to replace multiple words in a single line.
- Always print the result to verify the changes.
- Remember that the replace() method doesn’t alter the original string; it returns a new string.
Frequently Asked Questions
What if the word is not found in the string?
If the word is not found, the replace() method will simply return the original string unchanged.
Can I replace multiple words at once?
Yes, you can chain replace() methods, like my_string.replace("world", "Python").replace("Hello", "Hi")
.
Is the replace() method case-sensitive?
Yes, it is case-sensitive. "world" and "World" would be treated as different words.
Can I replace a word with an empty string?
Yes, you can replace a word with an empty string to remove it from the text, like my_string.replace("world", "")
.
How do I replace all occurrences of a word?
The replace() method replaces all occurrences of the word by default, so no additional steps are needed.
Summary
- Step 1: Open your Python environment.
- Step 2: Define your string.
- Step 3: Use the replace() method.
- Step 4: Save the new string.
- Step 5: Print the new string.
Conclusion
Replacing a word in Python is a straightforward task thanks to the built-in replace() method. By following the steps outlined in this guide, you can easily swap out words within any string. This method is not only efficient but also versatile, allowing for multiple replacements and case-sensitive adjustments. Whether you’re working on a small project or a large application, mastering this technique can enhance your text processing capabilities. For further reading, check out the official Python documentation on string manipulation. Now, go ahead and practice replacing words in Python to see how simple and effective it is!
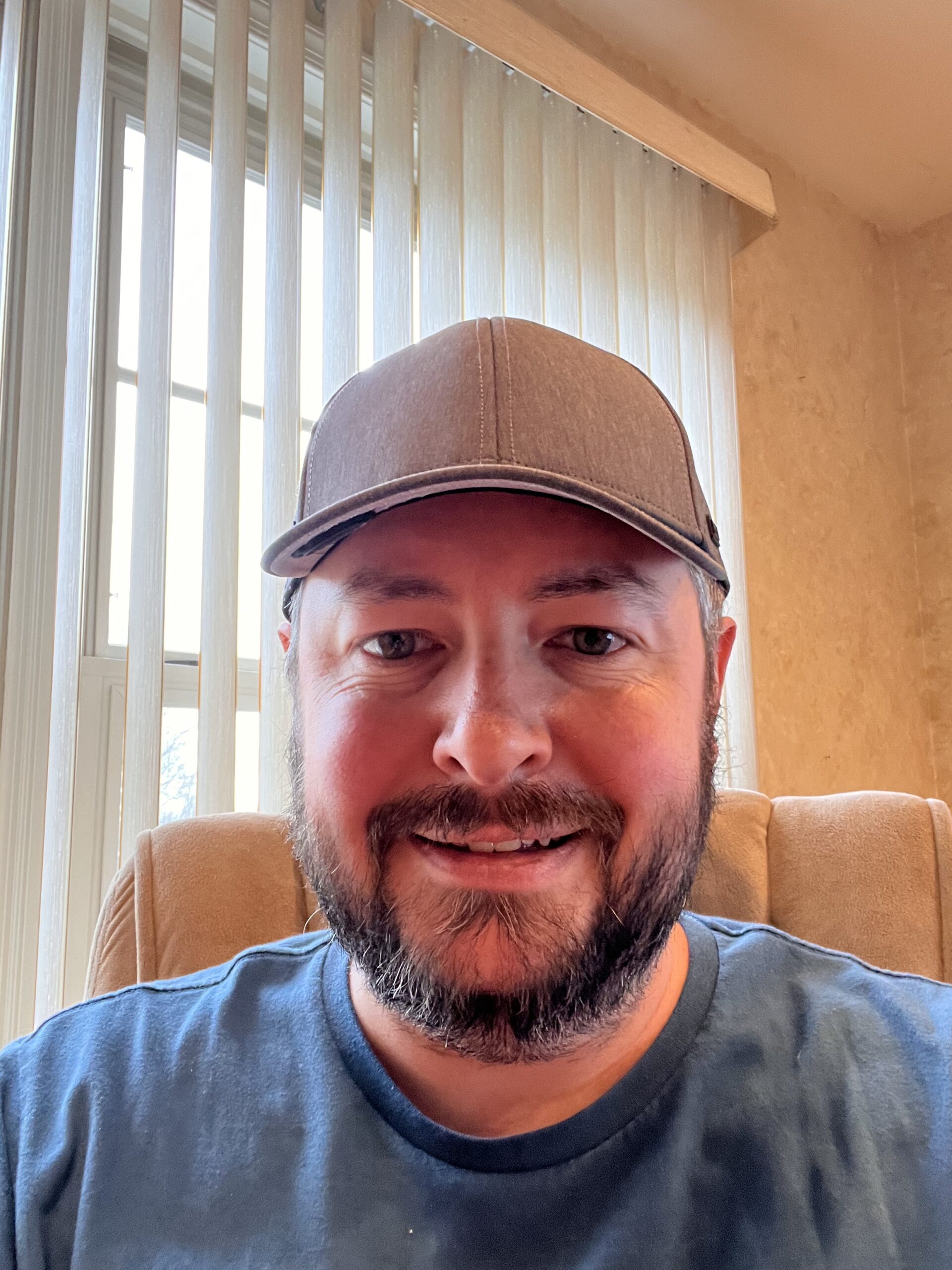
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.