Creating directories in Python is a piece of cake once you get the hang of it. Especially when dealing with non-existent paths, Python’s built-in libraries come to the rescue. You can breeze through the process with just a few lines of code. Ready to dive in? Let’s get those directories up and running!
Step by Step Tutorial: Creating Directories in Python
Before we get our hands dirty with code, let’s understand what we are about to do. We will create a directory (a fancy word for a folder) in Python. If the specified path doesn’t exist, we’ve got that covered too!
Step 1: Import the os module
First things first, you need to have the tools to do the job.
The os module in Python is like a Swiss Army knife for directory and path operations. It’s packed with handy functions that let us interact with the operating system.
Step 2: Define the path
Next up, tell Python where to create the new directory.
A path is simply the address where the directory will live on your computer. It’s like telling a taxi driver where to go – you need to give clear directions, or you might not end up where you want!
Step 3: Check if the path exists
Before creating anything, we need to see if the path is already there.
Checking if a path exists is crucial. It’s like looking both ways before crossing the street – it prevents accidents, or in our case, errors!
Step 4: Create the directory
Time to bring the directory into existence!
Creating the directory is the moment of truth. It’s like planting a seed in the ground – with a bit of care (and code), it will grow into a useful folder for your files.
After following these steps, you’ll have a brand-new directory ready to use. If the path didn’t exist before, Python will have created it for you. It’s like magic, but with code!
Tips: Creating Directories in Python
- Always use the os module – it’s your best friend for managing paths and directories.
- Check if the path exists before trying to create a directory, to avoid errors.
- Use descriptive names for your paths and directories, so you know what’s what.
- Remember that paths are different on Windows and Unix systems – keep this in mind if your code needs to be cross-platform.
- Experiment with different functions in the os module to see what else you can do with directories.
Frequently Asked Questions
What is a directory in Python?
A directory is a folder on your computer where you can store files. It’s like a drawer in a filing cabinet.
Can I create a directory with a non-existent path?
Yes, Python’s os module allows you to create directories at paths that don’t exist yet. It’s like building a house on an empty lot.
What is the os module in Python?
The os module is a built-in Python library that lets you interact with the operating system. It’s like having a remote control for your computer’s files and directories.
What happens if I try to create a directory that already exists?
Python will throw an error if you try to create a directory that exists unless you tell it not to. It’s like trying to build a house where one already stands.
How do I check if a path exists in Python?
You can use the os.path.exists() function to check if a path exists. It’s like having a map to see if you’re going to the right place.
Summary
- Import the os module.
- Define the path.
- Check if the path exists.
- Create the directory.
Conclusion
Creating directories in Python is a fundamental skill that can make organizing files and writing more complex programs a breeze. By harnessing the power of the os module, you have a robust toolkit for handling non-existent paths and ensuring your directories are set up just right. Whether you’re a beginner or a seasoned coder, mastering this task will serve you well in your programming endeavors.
Remember, the key is to be methodical and use the tools Python provides to your advantage. Always check if a path exists before creating a directory, use clear and descriptive names, and keep in mind the differences in file systems across platforms. With these tips in mind and a bit of practice, you’ll be creating directories like a pro in no time.
For those looking to expand their Python skills, diving deeper into the os module and exploring its other functionalities is a great next step. There’s a whole world of file and directory manipulation awaiting your discovery. So go ahead, give it a try, and see what amazing things you can build with your newfound knowledge of creating directories in Python!
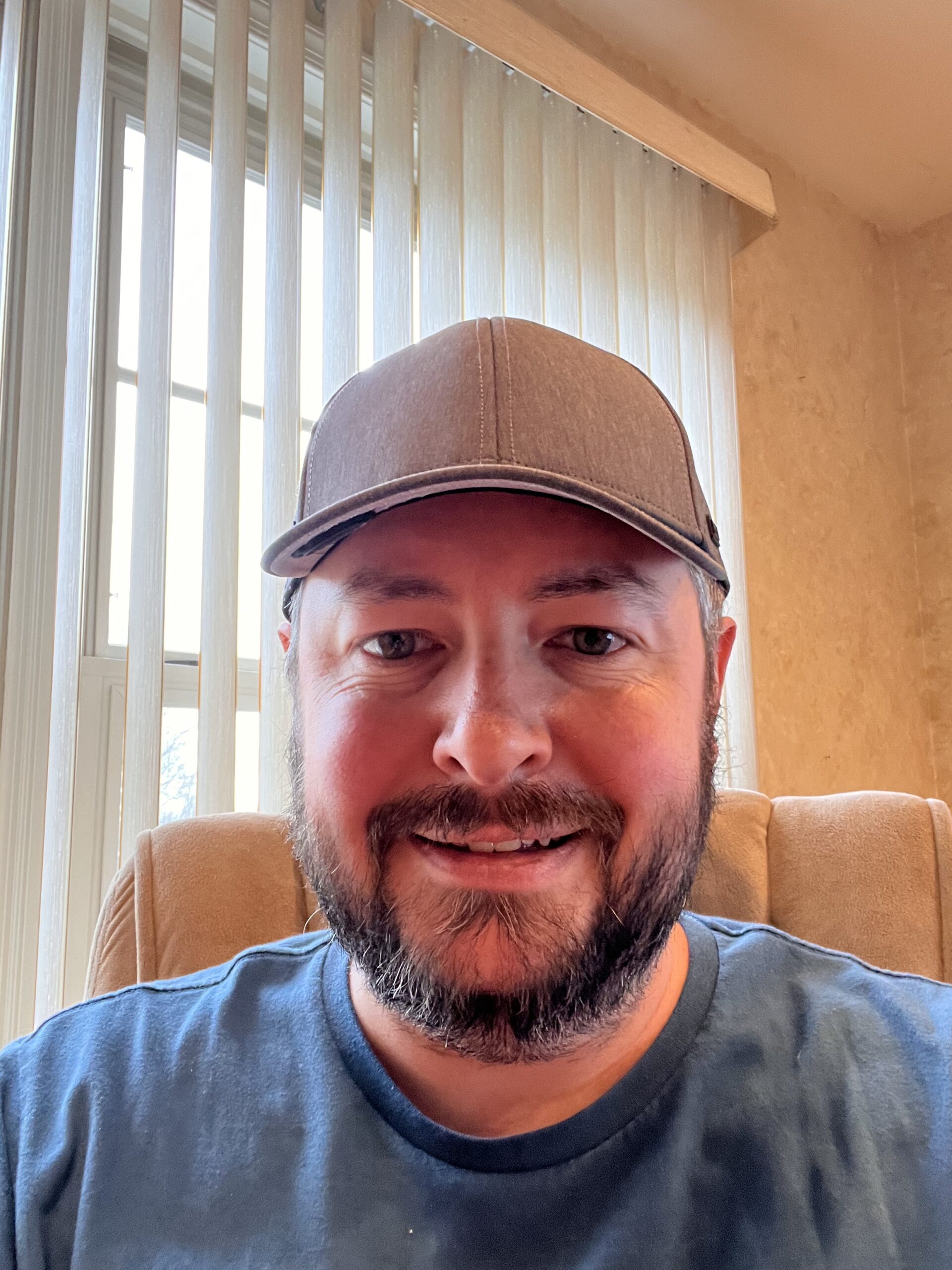
Kermit Matthews is a freelance writer based in Philadelphia, Pennsylvania with more than a decade of experience writing technology guides. He has a Bachelor’s and Master’s degree in Computer Science and has spent much of his professional career in IT management.
He specializes in writing content about iPhones, Android devices, Microsoft Office, and many other popular applications and devices.